Beyond Hoisting: Mastering the TDZ in JavaScript
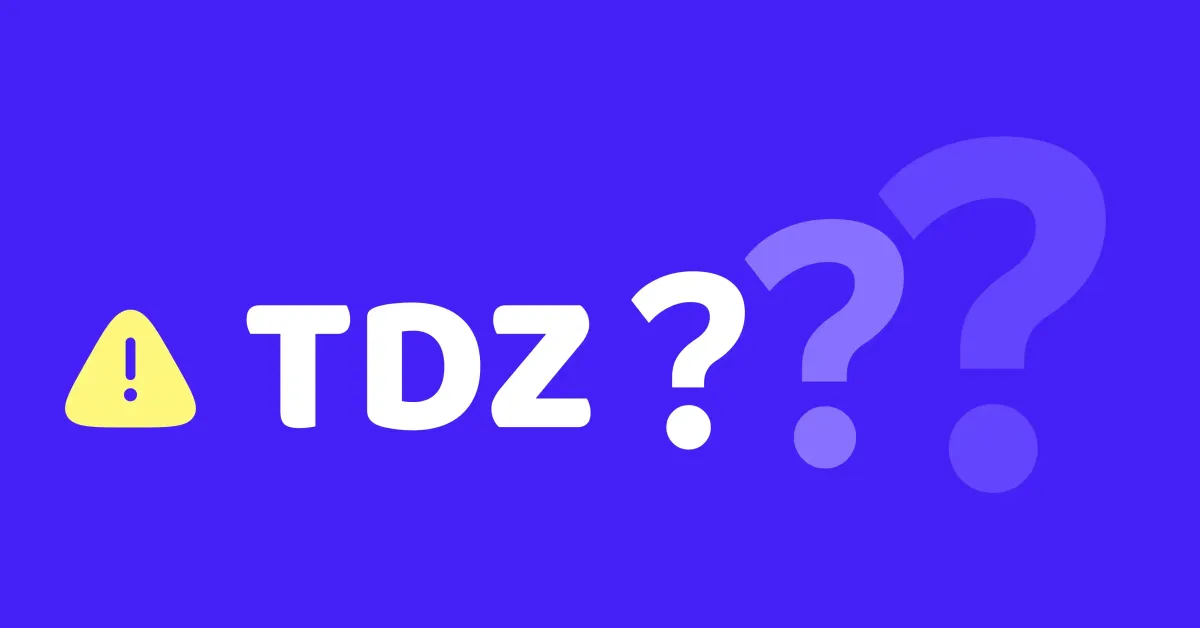
If you’ve worked with JavaScript, you’re probably familiar with the differences between let
, const
, and var
. But there’s a term you might not have come across: TDZ or Temporal Dead Zone.
According to the concept of hoisting, JavaScript places all variable and function declarations at the beginning of their respective scopes at the start of execution. However, it’s important to note that hoisting only applies to declarations and not to the initialization of any variable.
#Here’s how var
is treated differently compared to let
and const
:
#var
:
- When the JavaScript engine applies hoisting, the
var
declaration is lifted to the top of its scope, and the engine immediately assignsundefined
to thatvar
. - When the engine reaches the initialization statement, it assigns the actual value to the
var
. If no value is found, it remainsundefined
.
console.log(name); // -> undefined (because of hoisting)
function foo() { // core...}
var name;
console.log(name); // -> Ahmed
name = "Ahmed";
#let
and const
:
- When the JavaScript engine applies hoisting, the
let
declaration is lifted to the top of its scope, but the engine doesn’t assign any value at this point. - The engine assigns the actual value only when it reaches the initialization statement.
console.log(name); // -> ⚠️ Reference Error.
function foo() { // core...}
let name;
console.log(name); // -> Ahmed
name = "Ahmed";
The difference with
const
is that it prevents reassignment or redeclaration after its initial assignment.
If you try to access a var
before its declaration, JavaScript will return undefined
. However, if you try to access a let
or const
before their declarations, you’ll get a Reference Error.
This leads to a specific area in your code where you can’t access any let
or const
variable. This area starts from the beginning of the scope and ends at the start of the declaration statement.
/* Start of TDZ */
console.log(name); // -> ⚠️ Reference Error.
/* End of TDZ */let name;
console.log(name); // -> Ahmed
name = "Ahmed";
This is what’s known as the TDZ.